Last time I got a LoRa Server and LoRa Client to communicate to each other. It was about time to give them something to talk about i.e. start exchanging sensor data rather than just “Hello World”.
RFID tags
I plugged in my RFID reader which I bought like a years ago. My Arduino UNO was getting scrambled data on its serial. I tried connecting the RFID reader alone. The Arduino detected the device but whenever I’d place an RF tag on it, no data would be read.
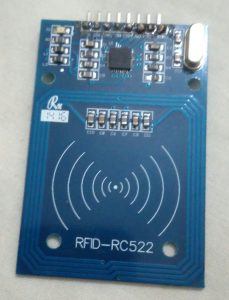
The forums suggested to solder the pins on the board for better connection but it didn’t work in my case. Most probably my device was faulty.
Fortunately my friend Damien from DM Electronics had the device is his stock which he sold to me. I’d highly recommend you to buy your electronic devices from there if you’re in Mauritius. He’s an awesome guy who has a deep understanding about how the devices actually operate on the inside.
https://www.youtube.com/watch?v=23aMjljCLZI
I followed the tutorial above with Damien’s RC522 and it worked. But this is only half of my problems. I still need to put the LoRa shield back on.
Making LoRa and RFID reader work together
The LoRa shield and RC5222 use Serial Peripheral Interface (SPI) to communicate with the Arduino. SPI was new to me since I was familiar with 3 pin sensors (Ground, 3.3V and Data). SPI consists of:
- 3.3V: Provides power. Easy
- Ground: Well, ground
- RST: Reset: Still no idea what it does. Can you tell me in the comments?
- SS: Slave Select: It sends a signal when the device shall listen and transmit data.
- MOSI: Master Out Slave In: The line which is used to receive data from the Arduino
- MISO: Master In Slave Out: The line which is used to send data to the Arduino.
Since the SS line exists, it should be possible to mute the RFID reader while LoRa is transmitting/receiving data. The MFRC522 library allows the user to set a custom SS and RST pin.
#define RST_PIN 6 #define SS_PIN 7 MFRC522 mfrc522(SS_PIN, RST_PIN);
The default 9,10 pins will be used by LoRa and 6,7 pins will be used by RFID Reader. I tried manually setting turning of LoRa shield by setting the SS pin high without success
pinMode(10, OUTPUT); // sets the digital pin as output digitalWrite(10, HIGH);
Reading the source code of the SPI library (“SPI.h”), I came across the method `end()`. The RH_RF95 library also has a `sleep()` method. My final code looks like this:
void setup() { Serial.begin(9600); } void loop() { readCard(); delay(5000); } void readCard() { SPI.begin(); mfrc522.PCD_Init(); ... SPI.end(); lora(datafromcard); } void lora(String data) { rf95.init() ... rf95.send(data.c_str(), data.length()); ... rf95.sleep(); }
And there you go. LoRa and RFID working together.
This is a very nice description.
How can I contact you?
g.
Sir I need the difference between rf and lora based in advantages.